What is Web Development?
The process of designing and developing websites and online applications that are available via the Internet is known as web development and python is a programming language. It comprises designing, developing, and maintaining websites with a range of functions and uses by utilizing a variety of technologies, programming languages like python, and tools.
Web development fundamentally consists on two components:
- Front-end development
- Back-end development.
The visual and interactive components of a website that visitors interact with directly are the focus of front-end development. Using tools like HTML (Hypertext Markup Language), CSS (Cascading Style Sheets), and JavaScript, this entails developing the layout, designing the user interface (UI), and implementing the visual components. Websites that are aesthetically pleasing, responsive, and user-friendly are made possible by front-end developers.
On the other hand, back-end development works with a website’s server-side functionality. It entails developing the architecture and logic needed to process data, manage database interactions, and maintain the operation of the website. Programming languages like Python, Ruby, Java, or PHP are used by back-end developers to create the server-side parts of websites.
What is Python Web Development?
The process of developing websites and web applications with the Python programming language is known as Python web development. Python is a widely used and adaptable programming language that is well-known for its ease of use, readability, and extensive library and framework ecosystem.
Web developers write the server-side logic in Python that powers online applications. This involves managing HTTP requests and responses, storing and retrieving data, presenting dynamic content, and carrying out business logic.
Why use Python for Web Development?
Python is a well-liked programming language that has become rather prominent in the web development industry. It is a great option for creating scalable and reliable web applications because it has several benefits. The following are some strong arguments in favor of Python’s widespread usage in web development:
- Readability and Simplicity: Python’s easy-to-read and write syntax highlights the readability and maintainability of code. Developers may express concepts in fewer lines of code thanks to its straightforward syntax, which expedites and boosts development productivity. Python’s user-friendliness allows experienced and inexperienced engineers to collaborate effectively.
- Large and Active Community: The Python community is big and active, with lots of developers helping to shape it and contribute to its development. The community offers a wealth of frameworks, tools, and resources created specifically for web development. Python’s abundance of community-driven tools and resources, which address a wide range of demands, make it a compelling choice for web development.
- Extensive Libraries and Frameworks: Python’s extensive ecosystem of modules and frameworks simplifies web development jobs. Django, one of the most popular Python web frameworks, provides a robust and complete toolbox for building complex web applications. Model-View-Controller (MVC) architecture is followed, and features like authentication, database ORM (Object-Relational Mapping), and URL routing are supported straight out of the box. Another lightweight, flexible micro-framework that gives developers more flexibility over the components and structure of their applications is Flask. These frameworks, along with others like Pyramid and Bottle, provide a solid foundation and increase the productivity of web development.
- Scalability and Performance: Python is renowned for its scalability and performance, which makes it perfect for handling high traffic online applications. Thanks to advancements like asynchronous programming, Python frameworks like Django and asyncio can efficiently manage concurrent requests and maximize server resources. Additionally, Python’s integration features simplify language integration, enabling programmers to leverage high-performance C or C++ libraries as needed.
- Integration and Compatibility: Python can easily integrate with other technologies and is flexible enough for web development. Numerous database types are supported, including SQL-based databases like MySQL, PostgreSQL, and SQLite as well as NoSQL databases like MongoDB. Python allows developers to easily integrate different components and services since it works with web servers, message queues, caching systems, and APIs.
- Testing and Debugging: Python boasts robust testing frameworks like unittest and pytest that simplify the process of creating and executing tests for online applications. Integrated development environments (IDEs) and pdb are two of its debugging tools that help developers locate and fix issues more quickly.
- Rapid Development: Python’s focus on simplicity and productivity allows developers to build web apps quickly. Developers can reuse pre-built modules and libraries to save time and effort by not having to start from scratch. For startups and small-scale endeavors, time-to-market is crucial, and this rapid development approach is highly beneficial.
How to use Python for Web Development
Python web application development can be accomplished with this flexible programming language. Python has gained popularity as a programming language for creating web applications because of its ease of use, readability, and extensive ecosystem of libraries and frameworks. The following are the essential steps to begin using Python for web development:
- Install Python: Start by installing Python on your machine. Visit the official Python website (https://www.python.org/) and download the latest version compatible with your operating system. Follow the installation instructions to complete the setup.
- Choose a Web Framework: Python provides a number of web frameworks that offer structures and tools to make web development more efficient. A few well-liked frameworks are Pyramid, Bottle, Flask, Django, and Pyramid. Look into and select a framework that fits the needs of your project and your level of experience.
- Set Up a Development Environment: Establish a virtual environment to separate your project’s dependencies and create a specific folder for it. Virtual environments are useful for managing packages particular to a project and avoiding problems with other Python installs. To construct and activate a virtual environment, you can use programs like virtualenv or Python’s built-in venv module.
- Install Framework and Dependencies: Once your virtual environment is activated, use the package manager pip to install the chosen web framework and any other required dependencies. For example, if you’re using Django, run “pip install Django” to install it.
- Project Initialization: Every web framework offers a unique method for establishing a brand-new project structure. For comprehensive instructions, see the documentation for the framework. To start a new Django project, use the command “django-admin startproject projectname” for Django.
- Configure Settings: The majority of web frameworks come with configuration files that let you set up things like database connections, static files, and security settings specifically for your project. Find the configuration file for the framework of your choice, then make the necessary changes.
- Define Models: Models show the links and structure in your data. Models are defined using a declarative syntax that corresponds to database tables in frameworks such as Django. To represent the data elements in your application, define your models.
- Create Views and Templates: Views handle the logic of processing requests and generating responses. Templates provide the structure and presentation of the web pages. Define your views to handle specific URL routes and connect them to corresponding templates.
- Define URL Routes:Set up the URL routing feature that your web framework offers. This associates incoming URLs with particular views or features in your application. To manage various routes, define URL patterns and indicate which view or function to call in response.
- Handle Forms and User Input: If your web application requires user input and form submissions, use the features provided by the framework to handle form processing, validation, and data persistence.
- Integrate with Databases: Database interaction is usually supported by default in Python web frameworks. Set up your database connection parameters in the framework’s setup file, then utilize ORM (Object-Relational Mapping) methods to connect Python code to the database.
- Implement Business Logic: Write the code required to put your web application‘s business logic into action. This could entail managing user identification, putting business rules into practice, handling data processing, and interacting with third-party services or APIs.
- Test and Debug: To make sure your web application is correct and functional, write tests. To make testing easier, the majority of Python frameworks come with testing tools and frameworks. During development, make use of debugging tools and procedures to find and address any problems or faults.
- Deploy and Maintain: When your web application is prepared, deploy it by selecting a suitable hosting environment. Make that the server environment satisfies the prerequisites and takes into account factors like scalability, performance optimization, and security. Maintain and update your application on a regular basis.
Different Python Web Development Frameworks
Python provides a number of web frameworks to meet a variety of purposes and tastes. Here are some popular Python web development frameworks:
- Django: High-level, feature-rich Django framework is well-known for its “batteries included” philosophy.It provides an extensive set of tools and features that enable the quick development of complex web applications. An ORM (Object-Relational Mapping) is built into Django and is used for form handling, database management, URL routing, authentication, and other purposes. It adheres to the architectural Model-View-Controller (MVC) pattern.
- Flask: Flask is a framework that is lightweight and adaptable; it is sometimes called a micro-framework. It gives developers more flexibility over the structure of their applications by providing the necessities for developing web applications in Python. While Flask can handle requests, render templates, and route URLs, it does not provide database administration or authentication; these are tasks best left to extensions and libraries. It is perfect for modest to medium-sized tasks and takes a minimalistic approach.
- Pyramid: Pyramid is a scalable and adaptable web framework that strives for power without sacrificing simplicity. It adheres to a minimalist concept and lets developers select the parts that they require. Pyramid has facilities for authentication, caching, and localization in addition to supporting a number of template engines and URL dispatching. It may be used for any size project, from little apps to complex business systems.
- Bottle: Bottle is a small-footprint, minimalist web framework. Because of its simple learning curve and ease of use, it is a great option for novices or small projects. Bottle offers basic HTTP request and response processing capabilities, template rendering, routing, and other features despite its simplicity. It is simple to deploy and distribute because it is a single-file module without any external dependencies.
- CherryPy: CherryPy is a streamlined web framework designed to be scalable, stable, and quick. It offers an easy-to-use API for managing sessions, routing URLs, and processing HTTP requests. CherryPy can be integrated with other servers or operated as a stand-alone HTTP server. Building small- to medium-sized applications and APIs is appropriate for it.
- Tornado: Tornado is a robust and expandable web framework that prioritizes performance and adeptly manages heavy traffic volumes. It allows non-blocking I/O operations and is intended for use in the construction of asynchronous web servers. Applications that need to operate in real-time, such chat servers and streaming platforms, can benefit from Tornado’s ability to manage thousands of connections at once.
These are just a few examples of Python web frameworks, and each has its own strengths and use cases. Consider your project requirements, scalability needs, learning curve, and community support when choosing the framework that best fits your development goals.
Python libraries for Web Development
Python web development provides a plethora of tools and frameworks to improve productivity and streamline the development process. The following libraries & tools are frequently used in Python web development:
- Requests: brary for requesting things over HTTP. It makes handling HTTP methods, headers, cookies, and authentication as well as dealing with web APIs easier.
- Beautiful Soup: A library for parsing XML and HTML is called Beautiful Soup. It is appropriate for web scraping and information extraction from web pages since it offers a straightforward API for examining and changing the parsed data.
- Pillow: Pillow is an effective image processing and modification package. It can be used to resize, crop, apply filters, and add text or overlays to photographs, among other things. The pillow is frequently used for managing and manipulating images in web applications.
- SQLAlchemy: A feature-rich ORM (Object-Relational Mapping) package called SQLAlchemy makes database administration in Python easier. Working with databases in web applications is made simpler by its support for numerous database engines and high-level API for database interaction.
- Celery: Celery is a distributed task queue library designed to allow web applications to execute tasks asynchronously. Your application will respond more quickly if you can assign labor- or resource-intensive tasks to be completed in the background.
- Flask-SQLAlchemy: An addon called Flask-SQLAlchemy combines SQLAlchemy with the Flask web framework. It facilitates easy database manipulation in Flask applications by offering seamless connection between Flask and SQLAlchemy.
- Flask-WTF: An extension for Flask applications that handles web forms is called Flask-WTF. It offers tools for managing form submissions, validating forms, and rendering forms. Managing user input and working with forms are made easier with Flask-WTF.
- PyJWT: A library for JSON Web Tokens (JWT) authentication is called PyJWT. It makes it easier to create, decode, and verify JWTs—which are frequently used in online applications for authorization and authentication.
- Redis-py: Redis is an in-memory data structure store; redis-py is a Python client for it. It enables you to work with Redis databases and carry out tasks like caching, pub/sub messaging, and data storing and retrieval.
- Pydantic: Pydantic is a module for data validation and parsing that makes working with complex Python data structures easier. It offers automatic data validation, serialization, and deserialization, and lets you design data models using type hints. Web applications frequently utilize Pydantic to handle and validate incoming request data.
- Jinja2: For Python, Jinja2 is a strong and adaptable template engine. It offers a syntax for creating templates that may be dynamically rendered with data and contain placeholders and logic. Web frameworks such as Flask and Django rely on Jinja2 extensively for the generation of HTML pages, emails, and other dynamic content.
These are only a handful of the numerous libraries for web development that are available in the Python environment. Explore and use these libraries to improve your web development workflow, based on the demands of your particular project.
A Roadmap for Web Development with Python
A roadmap for web development with Python that outlines the key steps and concepts involved in web development with Python:
- Learn the basics of Python: Learn the syntax, data types, control structures, and functions that are essential to Python programming. To begin started, you can consult books or internet guides.
- Understand HTML, CSS, and JavaScript: Acquire a fundamental understanding of web technologies, such as JavaScript for client-side interactivity, CSS for styling, and HTML for markup. These are necessary to comprehend and design web sites.
- Choose a web framework: Choose a Python web framework based on the needs of your project. A few well-liked choices are Pyramid, Flask, and Django. To make an informed choice, review the documentation and available resources for each framework. Each has unique capabilities and a unique learning curve.
- Front-end development: Develop your web development abilities by being familiar with well-known front-end frameworks and modules like Angular, Vue.js, and React. You may create interactive user interfaces and interfaces with back-end APIs using these frameworks.
- RESTful API development: Study RESTful design patterns and principles if your project involves developing an API. Create APIs that provide data and functionality to other apps or front-end interfaces using the web framework of your choice.
- Authentication and authorization: Recognize the meanings of authorization and user authentication. Learn how to use the built-in capabilities or extensions of your web framework to develop secure user registration, login, and access control techniques.
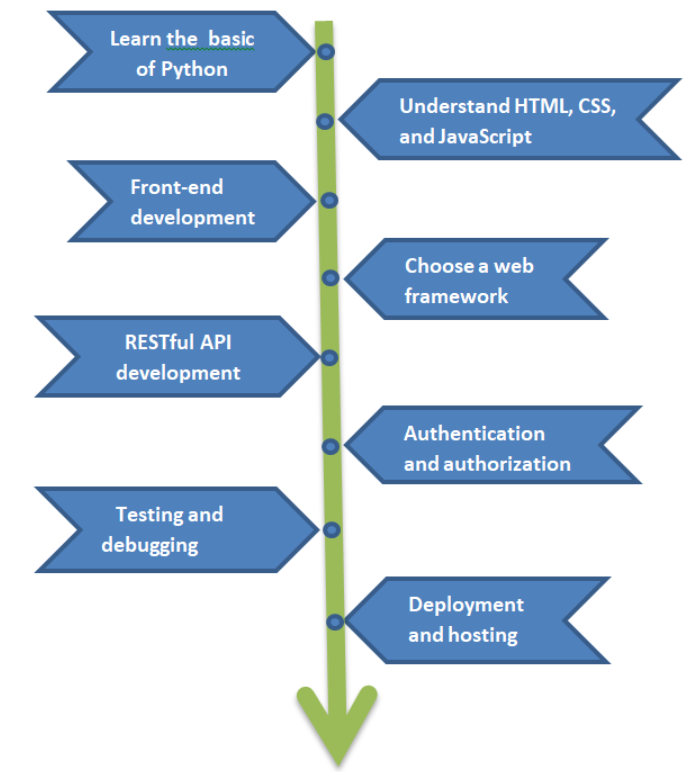
- Testing and debugging: Gain proficiency in testing your web applications. Learn about unit testing, integration testing, and end-to-end testing. Use tools like pytest, Selenium in Python, or the testing frameworks provided by your chosen web framework to write and execute tests.
Read More: Top 8 Python Testing Frameworks in 2023
- Deployment and hosting: Find out how to put your web application on a cloud platform or web server. Gain an understanding of ideas like scalability, security considerations, deployment automation, and server setup. For hosting web applications, platforms like Heroku, AWS, or PythonAnywhere are frequently utilized.
Keep in mind that this roadmap is only a broad sketch; you can modify it to fit the needs and preferences of your project. To bolster your comprehension and acquire real-world experience, it is critical to work on practical projects and investigate real-world examples.
How to create your first web application in Python
To create your first Python web development example, Need to follow the below steps:
- Install Python: Make sure Python is installed on your computer. The most recent version is available for download from the official Python website. (https://www.python.org).
- Choose a Web Framework: Choose a web framework based on what you require. Django and Flask are well-liked options for novices. For this example, Flask will be used.
- Install Flask: Run the following command at the command prompt or terminal to install Flask using pip, a Python package installer:
pip install flask
- Create a Project Folder: Create a new folder for your project. This will be the root directory for your web application.
- Create a Python File: Inside your project folder, create a new Python file. For example, app.py.
- Import Flask and Create an App Instance: In app.py, import the Flask module and create an instance of the Flask class. Add the following code in the app.py file:
from flask import Flask, render_template, request app = Flask(__name__)
- Define a Route and View Function: Define a route (URL) and a view function to handle the request. The view function will return the response that will be displayed in the browser. Add the following code:
@app.route('/login', methods=['GET', 'POST']) def login(): if request.method == 'POST': # Perform login authentication username = request.form['username'] password = request.form['password'] # Add your authentication logic here if username == 'admin' and password == 'password': return 'Login successful!' else: return 'Invalid username or password’ # If the request method is GET, render the login template return render_template('login.html')
- Create a Login HTML Template: Inside your project folder, create a new folder named templates. Inside the templates folder, create an HTML file named login.html. Add the following code to login.html:
<!DOCTYPE html> <html> <head> <title>Login</title> </head> <body> <h1>Login</h1> <form method="POST" action="/login"> <label for="username">Username:</label> <input type="text" id="username" name="username" required><br><br> <label for="password">Password:</label> <input type="password" id="password" name="password" required><br><br> <input type="submit" value="Login"> </form> </body> </html>
- Run the Application: At the end of app.py, add the following code to run the Flask application:
if __name__ == '__main__': app.run()
- Start the Development Server: Before start server, we need to compile above steps code together, Here is an entire script for app.py file :
from flask import Flask, render_template, request app = Flask(__name__) @app.route('/login', methods=['GET', 'POST']) def login(): if request.method == 'POST': # Perform login authentication username = request.form['username'] password = request.form['password'] # Add your authentication logic here if username == 'admin' and password == 'password': return 'Login successful!' else: return 'Invalid username or password' # If the request method is GET, render the login template return render_template('login.html') if __name__ == '__main__': app.run()
In your terminal or command prompt, navigate to the project folder and run the following command:
python app.py
- Open the Login Page: Open your web browser and enter http://localhost:5000/login in the address bar. You should see a login page with a username and password field.
- Test Login Functionality: Enter “admin” as the username and “password” as the password, and click the “Login” button. You should see a “Login successful!” message. If you enter any other username or password combination, you will see an “Invalid username or password” message.
This example shows how to use Flask to create a simple login page. Depending on the needs of your project, you can build on this by including database integration, user authentication, session management, and other security features.
Best Practices for Python Web Development
There are a few best practices for Python web development that you can adhere to in order to produce code that is clear, effective, and manageable. Here are six crucial things to think about:
- Use a Web Framework: Python offers a wide range of web frameworks like Django, Flask, and Pyramid. These frameworks provide essential tools and features for web development, such as routing, request handling, and template engines. Choosing a framework helps you structure your codebase and promotes code reuse.
- Follow the MVC Pattern: Model-View-Controller (MVC) is a software architectural pattern commonly used in web development. It helps separate the concerns of your application by dividing it into three components: models (representing data and business logic), views (handling user interface), and controllers (managing the flow between models and views). Adhering to this pattern makes your code more modular and maintainable.
- Use Virtual Environments: Virtual environments create isolated Python environments for your projects, allowing you to manage dependencies and avoid conflicts between packages. Tools like virtualenv or Python’s built-in venv module enable you to create and activate virtual environments. This practice ensures project-specific dependencies and avoids cluttering your global Python installation.
- Employ Database Abstraction Layers: When working with databases, use an ORM (Object-Relational Mapping) library like SQLAlchemy. ORM libraries abstract the database layer, allowing you to interact with the database using Python objects and queries. This approach simplifies database operations, improves security by preventing SQL injection, and facilitates switching between different database systems.
- Write Unit Tests: Unit testing is crucial for maintaining code quality and catching potential bugs early. Python has a built-in testing framework called unittest, along with third-party libraries like pytest. Write comprehensive unit tests for your web application’s components, including models, views, and controllers. Automating tests helps ensure that future code changes don’t introduce unexpected issues.
- Handle Security: Web applications are vulnerable to various security threats. Some essential security practices include:
- Input Validation: Validate and sanitize all user inputs to prevent common attacks like SQL injection and cross-site scripting (XSS).
- Password Security: Store passwords securely using techniques like hashing and salting to protect user credentials.
- Cross-Site Request Forgery (CSRF) Protection: Implement measures like CSRF tokens to prevent unauthorized requests.
- Secure Session Management: To safeguard user sessions, use secure session management strategies including session timeouts and secure flagging in session cookies.
- Regularly Update Dependencies: Maintain the most latest versions of your Python packages and web frameworks to ensure you are running the most recent security upgrades.
These are only a few best practices to take into account while using Python to create web apps. Throughout your development process, do not forget to follow the guidelines for readability, maintainability, and scalability of code.
Conclusion
Python is a potent language that is well-suited for web development, which entails building websites and web apps. Because of its ease of use, readability, and robust environment, Python is a great option for web development projects. Python offers a strong framework for web development, allowing programmers to create feature-rich, scalable web apps quickly. Once your application is finished, you can use BrowserStack to test it end-to-end. It offers simultaneous test execution and support for a variety of browsers.